Understanding data structures and algorithms is crucial for anyone in the field of programming and software development. Data structures and algorithms are the building blocks of computer science and provide a systematic approach to problem-solving. Algorithms are like a set of instructions that a computer can follow to solve a problem, while data structures are containers that organize and manipulate data.
In order to master data structures and algorithms, it is important to start with a strong foundation in programming and learn a programming language like Java. Understanding the relationship between algorithms and data structures is also key, as the choice of data structure can greatly impact the performance of an algorithm.
The learning path for mastering data structures and algorithms involves starting with the basics of programming, then moving on to understanding different data structures such as arrays, linked lists, stacks, and queues. It is also important to master sorting and searching algorithms, as well as explore more advanced concepts like trees, graphs, and dynamic programming.
Practice is essential for mastery, so it is recommended to solve coding problems on platforms like LeetCode and participate in coding challenges. It is also beneficial to learn from others through textbooks, online courses, and participation in coding communities.
By mastering data structures and algorithms, individuals can enhance their problem-solving skills, write efficient code, and open up career opportunities in the field of software development.
Key Takeaways:
Practice is essential for mastering data structures and algorithms. Solving coding problems on platforms like LeetCode and participating in coding challenges can enhance problem-solving skills and reinforce understanding. It is also beneficial to learn from textbooks and online courses, which provide comprehensive resources for studying and deepening knowledge in these areas. Additionally, engaging in coding communities like forums, meetups, and online communities allows individuals to learn from others, share insights, and seek guidance.
By dedicating time and effort to mastering data structures and algorithms, individuals can enhance their problem-solving skills, write efficient code, and unlock a multitude of career opportunities in the field of software development. With these foundational skills, programmers can tackle complex challenges and contribute to the ever-evolving world of technology.
Before delving into data structures and algorithms, it is crucial to build a solid foundation in programming and acquire essential programming skills. A strong programming foundation provides the necessary knowledge and skills to understand and implement data structures and algorithms effectively.
To start, it is recommended to learn a programming language like Java, which is widely used in the industry. Java is known for its simplicity, readability, and versatility, making it an excellent choice for beginners. By mastering Java or any other programming language, individuals can gain a deep understanding of essential programming concepts like variables, loops, conditionals, and functions.
Additionally, it is important to practice writing code and develop problem-solving skills. By solving coding challenges and exercises, individuals can improve their logical thinking and algorithmic problem-solving abilities. Online platforms like Codecademy and HackerRank offer coding tutorials and challenges, providing valuable hands-on experience.
By gaining a deep understanding of the relationship between algorithms and data structures, you can make informed decisions when solving programming problems. It allows you to optimize the performance of your code and solve complex problems more efficiently. Additionally, a strong grasp of data structures and algorithms is highly valued in the tech industry and can open up numerous career opportunities in software development.
In the upcoming sections, we will delve into the various data structures and algorithms, from basic to advanced, providing you with the knowledge and skills to become a proficient programmer. We will explore sorting and searching algorithms, advanced data structures such as trees and graphs, and delve into problem-solving techniques. By following this learning path and practicing frequently, you will be well on your way to mastering data structures and algorithms.
Linked Lists: Linked lists consist of nodes that hold data and a reference to the next node. They offer dynamic size, efficient insertion and deletion, but slower access compared to arrays.
Stacks: Stacks follow the Last-In, First-Out (LIFO) principle and allow for adding elements to the top and removing elements from the top. They are commonly used for undo/redo operations, recursive function calls, and expression evaluation.
Queues: Queues follow the First-In, First-Out (FIFO) principle and allow for adding elements to the rear and removing elements from the front. They are frequently used in scenarios such as task scheduling, message passing, and breadth-first search.
By delving into these advanced data structures and algorithms, programmers can expand their problem-solving abilities and tackle more complex challenges. These concepts form the foundation for many advanced algorithms and can greatly enhance code efficiency and performance.
By solving coding challenges like this, we not only improve our problem-solving skills but also build a portfolio of solutions that showcase our abilities to potential employers. It demonstrates our proficiency in data structures and algorithms and sets us apart from other candidates in the competitive field of software development.
Remember, mastering data structures and algorithms is a lifelong journey, and it is important to continually update your knowledge as new algorithms and data structures emerge. By supplementing your practical learning with textbooks and online courses, you can enhance your understanding, strengthen your problem-solving skills, and stay ahead in the ever-evolving field of programming and software development.
Engaging in Coding Communities
Engaging in coding communities provides opportunities to learn from others, seek guidance, and share knowledge about data structures and algorithms. By joining coding forums, attending meetups, and participating in online communities, programmers can connect with like-minded individuals who are passionate about programming and software development.
In these communities, programmers can ask questions, seek help with coding challenges, and gain valuable insights from experienced practitioners. Sharing knowledge about data structures and algorithms allows individuals to learn new approaches, discuss best practices, and stay updated on the latest trends in the field.
Participating in coding communities also fosters a supportive environment where programmers can collaborate on projects, exchange ideas, and receive constructive feedback. By engaging with a diverse community, individuals can broaden their perspectives and discover innovative solutions to complex problems.
Overall, joining coding communities is a valuable resource for anyone looking to enhance their understanding of data structures and algorithms. It provides a platform for continuous learning, professional development, and networking, ultimately contributing to the growth and success of programmers in the field of software development.
Unlocking Career Opportunities
Mastering data structures and algorithms opens up numerous career opportunities in the field of software development. In today’s technology-driven world, where data and information are constantly growing, the ability to efficiently organize, process, and analyze data is in high demand. As a result, companies are seeking professionals who have a strong foundation in data structures and algorithms to optimize their systems for maximum performance and scalability.
Professionals with a deep understanding of data structures and algorithms are highly sought after by top tech companies, startups, and IT organizations. They play a crucial role in designing and implementing efficient software solutions, solving complex problems, and improving system performance. Whether it’s building robust web applications, developing cutting-edge artificial intelligence algorithms, or optimizing database management systems, the knowledge and skills gained from mastering data structures and algorithms are invaluable.
With the continuous growth of the tech industry, there is a constant need for skilled professionals who can tackle the ever-evolving challenges in software development. By mastering data structures and algorithms, individuals can position themselves for a wide range of job prospects, including software engineer, data analyst, system architect, and algorithm developer. These roles often come with competitive salaries, benefits, and opportunities for professional growth and advancement.
In conclusion, mastering data structures and algorithms not only enhances problem-solving skills and code efficiency but also unlocks a world of career opportunities in the field of software development. By continually learning, practicing, and staying updated with the latest advancements, individuals can position themselves as valuable assets in the tech industry and contribute to the development of innovative solutions that shape our digital world.
Continual Growth and Improvement
Continual growth and improvement in data structures and algorithms are crucial for staying competitive in the ever-evolving field of software development. As technology advances and new challenges arise, it is essential for programmers to expand their knowledge and skills in order to tackle complex problems efficiently.
To ensure continual growth in data structures and algorithms, it is important to stay updated with the latest advancements and developments in the field. This can be achieved by regularly reading research papers, following industry experts, and participating in online forums and communities dedicated to discussing cutting-edge algorithms and data structures.
Exploring New Algorithms and Data Structures
One effective way to continue improving in data structures and algorithms is to explore new concepts and algorithms beyond the basics. By delving into advanced data structures such as trees, graphs, and dynamic programming, programmers can broaden their understanding and enhance their problem-solving skills.
Additionally, experimenting with different algorithms and data structures in various scenarios can help programmers gain valuable insights and discover optimal solutions. This can be done through hands-on coding exercises, participating in coding challenges, and working on real-world projects that require efficient algorithmic solutions.
Continuous Practice and Learning
Continual growth in data structures and algorithms requires consistent practice and learning. Engaging in coding communities, participating in coding competitions, and solving coding problems regularly can help programmers sharpen their problem-solving skills and stay updated with the latest industry trends.
It is also advisable to learn from reputable textbooks and online courses dedicated to data structures and algorithms. These resources provide structured learning materials and exercises that facilitate understanding and mastery of important concepts.
By prioritizing continual growth and improvement in data structures and algorithms, programmers can stay competitive, write efficient code, and excel in the field of software development.
Conclusion
Understanding data structures and algorithms is a fundamental aspect of programming and software development, providing efficient problem-solving skills and opening up career opportunities. Data structures serve as the containers that organize and manipulate data, while algorithms act as the instructions that guide computers in solving problems.
To master data structures and algorithms, it is crucial to start with a strong foundation in programming. Learning a programming language like Java and acquiring essential programming skills will lay the groundwork for further exploration.
The relationship between algorithms and data structures is vital to optimize algorithm performance. Choosing the right data structure can significantly impact the efficiency of the algorithm, making it important to understand this connection.
Building a strong foundation in programming sets the stage for delving into basic data structures such as arrays, linked lists, stacks, and queues. Additionally, mastering sorting and searching algorithms is crucial for problem-solving efficiency. As individuals progress, exploring more advanced concepts like trees, graphs, and dynamic programming will enhance their knowledge in these areas.
Practice plays a key role in mastering data structures and algorithms. Engaging in coding challenges and solving problems on platforms like LeetCode are effective ways to enhance problem-solving skills. Additionally, learning from textbooks, online courses, and participating in coding communities provides valuable insights and guidance.
By mastering data structures and algorithms, individuals can elevate their problem-solving skills, write efficient code, and open up a wide range of career opportunities in the field of software development. Continual growth and improvement in these areas are crucial to stay updated with the latest advancements and maintain a competitive edge.
Understanding data structures and algorithms is not only a necessity but also a gateway to success in the ever-evolving world of programming and software development.
- Understanding data structures and algorithms is crucial for success in programming and software development.
- Data structures are containers that organize and manipulate data, while algorithms are a set of instructions for problem-solving.
- Choosing the right data structure can significantly impact the performance of an algorithm.
- A strong foundation in programming and continuous practice are essential for mastering data structures and algorithms.
- Exploring basic and advanced data structures, as well as sorting and searching algorithms, is vital for comprehensive knowledge.
Table of Contents
ToggleThe Role of Understanding data structures and algorithms in Problem Solving
Data structures and algorithms play a vital role in problem solving, with algorithms acting as instructions and data structures providing organization and manipulation capabilities. Understanding these concepts is crucial for anyone in the field of programming and software development. Algorithms serve as a set of instructions that guide computers in solving problems, while data structures act as containers that organize and manipulate data. In order to effectively solve problems, it is essential to choose the right data structure to optimize algorithm performance. The relationship between algorithms and data structures is symbiotic, as the choice of data structure can greatly impact the efficiency and effectiveness of an algorithm. By understanding this relationship, programmers can design solutions that are both elegant and efficient. Mastering data structures and algorithms requires a strong foundation in programming. Learning a programming language like Java can provide the necessary skills to explore and implement various data structures and algorithms. Starting with the basics of programming, individuals can gradually delve into more complex concepts like arrays, linked lists, stacks, queues, sorting algorithms, and searching algorithms. Building a solid understanding of these fundamental components is crucial for solving a wide range of problems.Benefits of Mastering Data Structures and Algorithms | |
---|---|
Efficient Problem Solving | With a strong understanding of data structures and algorithms, programmers can efficiently analyze problems and devise optimal solutions. |
Improved Code Quality | By leveraging the right data structures and algorithms, programmers can write code that is concise, readable, and performs well. |
Expanded Job Prospects | Proficiency in data structures and algorithms is highly valued in the tech industry, opening up a wide range of career opportunities in software development. |
Building a Strong Foundation in Programming
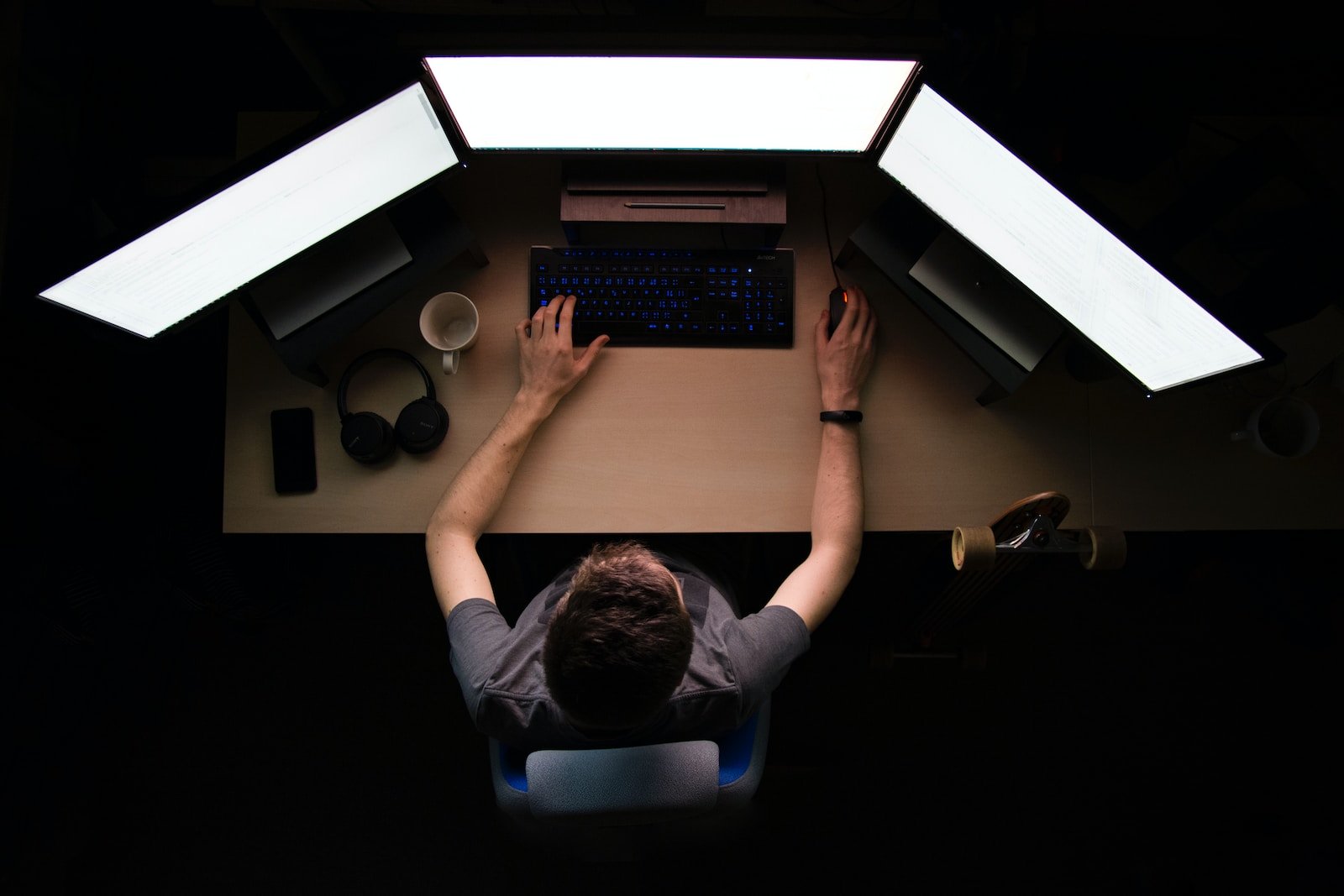
Essential Programming Skills:
- Understanding variables, data types, and control flow.
- Mastering loops and conditionals for iterative and conditional execution of code.
- Implementing functions and methods for code modularity and reusability.
- Understanding file handling and input/output operations.
- Practice problem-solving and algorithm development for efficient code implementation.
Programming Concepts | Description |
---|---|
Variables | Containers for storing data values. |
Data Types | Categories that define the type of data a variable can hold. |
Control Flow | Deciding the order in which statements are executed based on conditions. |
Loops | Repeating a set of instructions until a specific condition is met. |
Conditionals | Executing a set of instructions based on a condition. |
Functions | Reusable blocks of code that perform specific tasks. |
File Handling | Reading from and writing to files for data storage. |
Problem-Solving | Developing algorithms and logical thinking to solve complex problems. |
Understanding the Relationship Between Algorithms and Data Structures
To grasp the full potential of data structures and algorithms, it is vital to understand the relationship between them and how the choice of data structure affects algorithm performance. Algorithms are like a set of instructions that guide computers to solve problems, while data structures are containers that organize and manipulate data. The efficiency and effectiveness of an algorithm depend heavily on the data structure used. When designing an algorithm, choosing the right data structure can significantly impact its performance. For example, if you need to perform frequent insertions and deletions, a linked list may be more suitable than an array. Similarly, if you need to implement a search operation, a binary search tree can provide efficient lookup times compared to other data structures. By understanding the characteristics and operations of different data structures, you can optimize algorithm performance.Table: Common Data Structures and Their Characteristics
Data Structure | Characteristics |
---|---|
Array | Fixed size, random access |
Linked List | Dynamic size, efficient insertions and deletions |
Stack | Last-in, first-out (LIFO) structure |
Queue | First-in, first-out (FIFO) structure |
Exploring Basic Data Structures
In order to build a solid understanding of data structures and algorithms, it is essential to explore basic data structures such as arrays, linked lists, stacks, and queues. These fundamental data structures serve as the foundation for more complex ones and are widely used in various programming applications. Arrays: Arrays are a collection of elements of the same data type, stored in contiguous memory locations. They allow for efficient access and retrieval of elements using indices.Data Structure | Characteristics | Operations |
---|---|---|
Arrays | – Fixed size – Contiguous memory – Fast access | – Insertion – Deletion – Searching – Sorting |
Why Mastering Basic Data Structures Matters
Mastering these basic data structures is crucial as they form the building blocks for more complex ones and provide the necessary foundation to solve a wide range of programming problems efficiently. By understanding arrays, linked lists, stacks, and queues, programmers gain the ability to manipulate and organize data in a structured manner, leading to optimized algorithms and improved code performance. Moreover, a solid understanding of basic data structures enables programmers to approach more advanced concepts with confidence. Many advanced data structures, like trees and graphs, are built upon the principles and operations of these fundamental structures. By mastering the basics, programmers can easily grasp the intricacies of more complex data structures, expanding their problem-solving capabilities. In summary, mastering the basics of data structures such as arrays, linked lists, stacks, and queues is a crucial step in building a strong foundation in programming and problem-solving. These fundamental structures provide the necessary tools to manipulate and organize data efficiently, paving the way for more complex data structures and algorithms.Mastering Sorting and Searching Algorithms
Mastering sorting and searching algorithms is crucial for efficient problem solving, as these algorithms lay the foundation for various applications. Sorting algorithms are used to arrange data in a specific order, while searching algorithms help locate specific elements within a dataset. These algorithms play a vital role in optimizing code performance and improving overall efficiency. When it comes to sorting algorithms, there are various approaches available, each with its own advantages and disadvantages. Some commonly used sorting algorithms include:- Bubble sort
- Insertion sort
- Selection sort
- Merge sort
- Quick sort
Algorithm | Time Complexity |
---|---|
Bubble Sort | O(n^2) |
Insertion Sort | O(n^2) |
Selection Sort | O(n^2) |
Merge Sort | O(n log n) |
Quick Sort | O(n log n) |
Efficiency and Performance
Efficient sorting and searching algorithms can significantly improve the performance of software applications. By understanding the intricacies of these algorithms, developers can write code that processes data quickly and effectively. It is important to consider factors such as time complexity, space complexity, and the expected size of the dataset when choosing the appropriate algorithm. Additionally, understanding the limitations and trade-offs of each algorithm can help developers make informed decisions in real-world scenarios. Mastering sorting and searching algorithms is not only crucial for efficient problem solving, but it is also a key skill sought after by employers in the field of software development. Demonstrating a strong understanding of these algorithms can open up various career opportunities, as companies rely on efficient algorithms to handle large data sets, optimize code performance, and deliver high-quality software solutions.Diving into Advanced Data Structures and Algorithms
To delve deeper into data structures and algorithms, it is essential to explore more advanced concepts such as trees, graphs, and dynamic programming. These advanced data structures provide powerful ways to organize and manipulate data, allowing for more efficient problem-solving techniques.Advanced Data Structures
One of the key advanced data structures to study is trees. A tree is a hierarchical structure that consists of nodes connected by edges and is widely used for representing hierarchical relationships in computer science. Common types of trees include binary trees, AVL trees, and B-trees. Understanding different tree traversal algorithms, like preorder, inorder, and postorder, is crucial for navigating and manipulating tree structures. Graphs are another essential advanced data structure to explore. A graph is a collection of nodes, often referred to as vertices, connected by edges. Graphs can represent various relationships and are used in many real-world applications, such as network analysis and social network modeling. Understanding graph traversal algorithms, like breadth-first search (BFS) and depth-first search (DFS), is key to analyzing and manipulating graph structures. Dynamic programming is a powerful algorithmic technique used to solve optimization problems by breaking them down into smaller subproblems. It involves storing the solutions to subproblems in a table, which allows for more efficient computation of the overall problem. Dynamic programming can be applied to a wide range of problems, such as calculating Fibonacci numbers or finding the shortest paths in a graph.Advanced Data Structures | Applications |
---|---|
Trees | Representing hierarchical relationships |
Graphs | Network analysis, social network modeling |
Dynamic Programming | Solving optimization problems |
Practicing Problem Solving with Coding Challenges
Practice is key to mastering data structures and algorithms. Solving coding problems on platforms like LeetCode and participating in coding challenges enhances problem-solving skills. It allows us to apply the knowledge gained and develop a deeper understanding of how data structures and algorithms work in real-world scenarios. These coding challenges provide a variety of problems that test our ability to think critically and come up with efficient solutions. They cover topics ranging from basic data structures and algorithms to more complex problems, pushing us to expand our knowledge and skills. By actively engaging in coding challenges, we gain exposure to different problem-solving techniques and sharpen our analytical thinking. We learn how to approach problems systematically, break them down into smaller subproblems, and apply the appropriate data structures and algorithms to solve them.“The only way to really learn something is by doing it.”As we tackle more coding challenges, we also become better at optimizing our code for performance and efficiency. We learn to analyze the time and space complexity of our algorithms, ensuring that our solutions scale well with larger datasets.
Example of a Coding Challenge:
Challenge | Description |
---|---|
Two Sum | Given an array of integers, find two numbers that add up to a specific target sum. |
Sample Input | [2, 7, 11, 15], target = 9 |
Sample Output | [0, 1] (indices of the two numbers that add up to the target sum) |
Learning from Textbooks and Online Courses
Supplementing practical learning with textbooks and online courses is essential for a comprehensive understanding of data structures and algorithms. These resources provide in-depth explanations, examples, and exercises that reinforce the concepts learned through hands-on practice. Whether you prefer a self-paced online course or a traditional textbook, there are numerous options available to suit different learning styles and preferences. When choosing a textbook, look for one that covers the fundamentals of data structures and algorithms. Some recommended titles include “Introduction to Algorithms” by Cormen, Leiserson, Rivest, and Stein, as well as “Data Structures and Algorithms in Java” by Robert Lafore. These books are widely regarded as authoritative references in the field and offer clear explanations, code examples, and practice problems to solidify your understanding. If you prefer an interactive learning experience, online courses can be a valuable resource. Platforms like Coursera, Udemy, and edX offer a wide range of courses on data structures and algorithms, taught by experts in the field. These courses typically include video lectures, quizzes, coding assignments, and discussion forums, allowing you to learn at your own pace while still having access to support from instructors and peers.Benefits of Learning from Textbooks and Online Courses |
---|
Comprehensive Coverage: Textbooks and online courses provide a structured and organized approach to learning, covering all the necessary topics in data structures and algorithms. |
In-depth Explanations: These resources offer detailed explanations of concepts, algorithms, and data structures, helping you develop a deeper understanding of how they work. |
Practice Opportunities: Many textbooks and online courses provide exercises and coding assignments to reinforce your learning and allow for hands-on practice. |
Flexibility: Online courses can be accessed anytime and anywhere, allowing you to learn at your own pace and fit it around your schedule. |
Benefits of Engaging in Coding Communities | |
---|---|
Learning Opportunities | Gain knowledge and insights from experienced programmers. |
Guidance and Support | Get help with coding challenges and seek guidance from experts. |
Knowledge Sharing | Share your expertise and learn from others in discussions about data structures and algorithms. |
Networking | Connect with like-minded individuals and build professional relationships. |
Collaboration | Work together on projects, exchange ideas, and receive feedback. |
Career Opportunities | Description |
---|---|
Software Engineer | Design, develop, and maintain software applications using efficient algorithms and data structures. |
Data Analyst | Analyze and interpret complex data sets using algorithms and data structures to derive meaningful insights. |
System Architect | Design and optimize system architectures by utilizing data structures and algorithms for scalability and performance. |
Algorithm Developer | Create and optimize algorithms for various applications, such as machine learning, image processing, and optimization problems. |
Key Takeaways for Continual Growth in Data Structures and Algorithms: |
---|
Stay updated with the latest advancements and developments in the field through reading research papers, following industry experts, and participating in online communities. |
Explore advanced data structures and algorithms such as trees, graphs, and dynamic programming to broaden understanding and enhance problem-solving skills. |
Engage in continuous practice by participating in coding challenges, solving coding problems, and working on real-world projects. |
Learn from reputable textbooks and online courses dedicated to data structures and algorithms to gain structured knowledge and exercises. |
FAQ
Source Links
Why is understanding data structures and algorithms important?
Understanding data structures and algorithms is crucial for anyone in the field of programming and software development. They are the building blocks of computer science and provide a systematic approach to problem-solving.
What are data structures and algorithms?
Data structures are containers that organize and manipulate data, while algorithms are like a set of instructions that a computer can follow to solve a problem.
How can I master data structures and algorithms?
To master data structures and algorithms, it is important to start with a strong foundation in programming and learn a programming language like Java. Understanding the relationship between algorithms and data structures is also key.
What are some basic data structures to learn?
Some basic data structures to learn include arrays, linked lists, stacks, and queues.
Why should I learn sorting and searching algorithms?
Mastering sorting and searching algorithms is essential for efficient problem solving. They help in organizing and retrieving data effectively.
Are there more advanced data structures and algorithms to explore?
Yes, there are more advanced data structures and algorithms such as trees, graphs, and dynamic programming that you can dive into once you have a strong foundation.
How can I practice problem solving with coding challenges?
You can practice problem solving by solving coding problems on platforms like LeetCode and participating in coding challenges or contests.
What resources can I use to learn data structures and algorithms?
You can learn from textbooks, online courses, and tutorials that cover data structures and algorithms in depth.
How can engaging in coding communities help?
Engaging in coding communities allows you to learn from others, seek guidance, and share knowledge. It can provide valuable insights and support in mastering data structures and algorithms.
What career opportunities can mastering data structures and algorithms open up?
Strong knowledge in data structures and algorithms is highly desirable in the tech industry and can open up a wide range of job prospects in software development.